| |
Ejner
Registered: Oct 2012 Posts: 43 |
Show Koala Pic
I'm sure noone here has any problems with showing a simple Koala picture, but I came across this piece of code on codebase64.org and wondered why this had to be done so complicated...?
http://codebase64.org/doku.php?id=base:calculate_vic_settings_f..
In TurboAssembler it's pretty simple to calculate the VIC settings, see my example below. Isn't something similar possible with KickAssembler? Just wondering...
Example in TurboAssembler:
;---------------------------------------
; Show Koala
;---------------------------------------
koala = $6000
screen = $5c00
;---------------------------------------
bank = koala/$4000
*= $1000
lda #$3b
sta $d011
lda #koala-($4000*bank)/1024
ora #(screen-($4000*bank))/64
sta $d018
lda #$d8
sta $d016
lda $dd02
ora #%00000011
sta $dd02
lda $dd00
and #%11111100
ora #3-bank
sta $dd00
ldx #0
copy lda koala+$1f40,x
sta screen,x
lda koala+$2040,x
sta screen+$0100,x
lda koala+$2140,x
sta screen+$0200,x
lda koala+$2228,x
sta screen+$02e8,x
lda koala+$2328,x
sta $d800,x
lda koala+$2428,x
sta $d900,x
lda koala+$2528,x
sta $da00,x
lda koala+$2610,x
sta $dae8,x
inx
bne copy
lda koala+$2710
sta $d021
lsr a
lsr a
lsr a
lsr a
sta $d020
lda #0
cmp $0277 ;wait for key
bne *-3
lda $dd02
ora #%00000011
sta $dd02
lda $dd00
and #%11111100
ora #%00000011
sta $dd00
lda #$1b
sta $d011
lda #$15
sta $d018
lda #$c8
sta $d016
jmp $9000
;---------------------------------------
Note: The result of "bank = koala/$4000" is an integer. |
|
| |
Mace
Registered: May 2002 Posts: 1799 |
This shows how it's done in detail.
Of course for your own program, you can change it, but for educational purposes, it's sometimes easier to follow when everything is written in all detail. |
| |
Burglar
Registered: Dec 2004 Posts: 1101 |
.macro set_bank(screenram,bitmap) {
.var cur_dd00 = [ >screenram >> 6 ] ^ %00000011
.eval screenram = screenram & $3fff
.eval bitmap = bitmap & $3fff
.var cur_d018 = [ [ >screenram << 2 ] + [ >bitmap >> 2 ] ]
lda $dd00
and #%1111100
ora #cur_dd00
sta $dd00
lda #cur_d018
sta $d018
} and then you can :set_bank($0400,$2000) |
| |
Ejner
Registered: Oct 2012 Posts: 43 |
Thanks Burglar, thats a neat little snippet of code there :-) |
| |
Burglar
Registered: Dec 2004 Posts: 1101 |
annoying bug in the macro I posted. finally found out that *that* was causing random weirdness in 1 out of 20 runs or so.
lda $dd00
and #%11111100 <-- one more bit added
ora #cur_dd00
sta $dd00
subtle type, I was anding with #$7c instead of #$fc. it will bug your loader possibly. also might add that even this fixed one isnt 100% stable if you use it while loading |
| |
Slammer
Registered: Feb 2004 Posts: 416 |
Burglars way of doing it is pretty handy.. Especially when setting dd00. An alternative way of doing these things are defining functions like:
.function screenToD018(addr) {
.return [[addr&$3fff]/$400]<<4
}
.function charsetToD018(addr) {
.return [[addr&$3fff]/$800]<<1
}
.function toD018(screen, charset) {
.return screenToD018(screen) | charsetToD018(charset)
}
.function toSpritePtr(addr) {
.return [addr&$3fff]/$40
}
etc.
With functions like these you can do:
lda #toD018(logoScreen, logoCharset)
sta $d018 but also make tables if thats what you need:
.byte toD018(screen1,charset), toD018(screen2,charset)
So here you gain a little flexibility but have to type some more |
| |
SIDWAVE Account closed
Registered: Apr 2002 Posts: 2238 |
as the book says:
selectchar
lda $d018
and #240
ora #0 ;0-2-4-6-8-10-12-14 : 0000-07ff, 0800-0fff, 1000-17ff, 1800-1fff
; 2000-27ff, 2800-2fff, 3000-37ff, 3800-3fff
sta $d018 |
| |
Ejner
Registered: Oct 2012 Posts: 43 |
Hey, I see this thread is still cooking :-)
Thanks for all the examples, they are really helpful and give inspiration for digging a little deeper into all the smooth possibilities with f.i. KickAssembler, it seems like a really handy tool with lots of flexibility once you get the hang of it :-)
I think this thread now provides some nice examples that are all much smoother ways of calculating the VIC settings than the codebase example (no offence)...
Trying to learn a little from the examples above, does this compute in KickAssembler terminology?
koala = $6000
screen = $5c00
*= $1000
lda #[koala & $3fff]/1024
ora #[screen & $3fff]/64
sta $d018
lda $dd00
and #%11111100
ora #3-[>koala >> 6]
sta $dd00
|
| |
SIDWAVE Account closed
Registered: Apr 2002 Posts: 2238 |
this is unreadable code:
lda #[koala & $3fff]/1024
ora #[screen & $3fff]/64
sta $d018
lda $dd00
and #%11111100
ora #3-[>koala >> 6]
sta $dd00
lda d018
ora xxx
sta d018
can be read!
lda dd00
and xxx
ora 0-1-2-3
sta dd00
can be read
i tried kickasm.. its russian gibberish to me :) |
| |
Ejner
Registered: Oct 2012 Posts: 43 |
koala = $6000
screen = $5c00
*= $1000
lda #[>koala & $3f]/4
ora #[screen & $3fff]/64
sta $d018
lda $dd00
and #%11111100
ora #3-[>koala >> 6]
sta $dd00
Breaking it down...
lda #[koala & $3fff]/1024
...will take the address of the koala, then AND it with $3fff to skip the first two bits which define the VIC bank, and then divide this with 1024, which is actually LSR'ing 10 times to get the remaining bits in the right location for $d018. Treatet as a word, but the result is a byte.
ora #[screen & $3fff]/64
...same, but will LSR only 6 times.
ora #3-[>koala >> 6]
...">koala" will take only the highbyte of the koala address. Then it is LSR'ed 6 times, leaving only the two bits that define the VIC bank. "3-" will invert those two bits.
Hope this helps for better understanding... |
| |
chatGPZ
Registered: Dec 2001 Posts: 11386 |
hooray for using proper constants (and dont listen to rambones, hardcoding values is never better)
just one thing - why doing LDA# followed by ORA#? you can just write it like
Quote:
lda #[[>koala & $3f]/4]|[[screen & $3fff]/64]
also, use bitshifts instead of divides, it makes it easier to see what happens (IMHO) |
| |
soci
Registered: Sep 2003 Posts: 480 |
From the calculations above it might not be obvious but the same address lines are wired to the top and bottom nibble of $d018.
Bit 0 could have been used for finer font base address selection in ECM mode, unfortunately that's not how it was implemented.
=8192 bitmap = 8192
=1024 screen = 1024
.block
=$2000 bmpfont .var $0000 | bits(bitmap)
=$0400 screen .var $0000 | bits(screen)
.0000 a9 18 lda #$18 lda #screen[10:14]..bmpfont[10:14]
.0002 8d 18 d0 sta $d018 sta $d018
.0005 ad 00 dd lda $dd00 lda $dd00
and #~(screen[14:16] || [])
.0008 09 03 ora #$03 ora #(~screen)[14:16] || []
.000a 8d 00 dd sta $dd00 sta $dd00
.cerror (screen ^ bmpfont)[14:16], "different bank?!"
.bend
|
| |
Ejner
Registered: Oct 2012 Posts: 43 |
Groepaz, soci:
Okay, I didn't know that "|" (ORA?) feature before. I learn something new all the time :-)
koala = $6000
screen = $5c00
*= $1000
lda #[[>screen & $3f] << 2] | [[>koala & $3f] >> 2]
sta $d018
lda $dd00
and #%11111100
ora #3-[>koala >> 6]
sta $dd00
|
| |
Oswald
Registered: Apr 2002 Posts: 5094 |
soci, thats a neat idea, tho I cant think of a scenario where it would be useful :) maybe some demo effect with lots of prepped csets. |
| |
Dr.j
Registered: Feb 2003 Posts: 277 |
Soci , hehe i don't like to kill a fly with RPG but
what do you mean
by:
$0000 | bits(bitmap) where is the bits function? or i miss something.
and later:
and #~(screen[14:16] || []) hehe for not a C coders
could you make a friendly explain ? (you can do it on
private if you prefer) |
| |
Flavioweb
Registered: Nov 2011 Posts: 463 |
I think Soci is talking about 64tass:
http://tass64.sourceforge.net/ |
| |
Dr.j
Registered: Feb 2003 Posts: 277 |
oh Flavioweb ok. i am kickass person so i leave it aside
for now hehe |
| |
soci
Registered: Sep 2003 Posts: 480 |
Too much late coding yesterday ;) It would go even more off topic if I'd go into details in this thread.
The code fragment was only a demonstration about constructing $d018 values without using divisions and shifts.
Normally I use some variant of this:
lda #((font >> 10) & $0f) | ((screen >> 6) & $f0)
sta $d018
lda $dd00
ora #3
eor #font >> 14
sta $dd00 Even if this version is wasting 2 bytes half the time for the $dd00 calculation. |
| |
chatGPZ
Registered: Dec 2001 Posts: 11386 |
why not just store a fixed value to $dd00 though? i dare to say that "properly" ORing the value is very rarely needed - certainly almost never in the usual setup code at the start of your intro/demo/whatever :) |
| |
soci
Registered: Sep 2003 Posts: 480 |
Not sure why. I just checked and even my one filers are mostly doing the masking, where there's no chance of loader interference.
It must be tradition then.
So I now declare trademark rights on the ora/eor version. It may only identify genuine Singular productions ;) |
| |
chatGPZ
Registered: Dec 2001 Posts: 11386 |
i see this often, also with eg $01 or $d011 .... people must have learned it somewhere :) |
| |
The Phantom
Registered: Jan 2004 Posts: 360 |
Why not give an example of code? Unabridged CODE. No documentation per line of code, just CODE.
Screen Ram - $7f40-$8327 ?
Color Ram - $8338 (or is it $8328)-$8711?
My code, and it doesn't display proper at all.. Like missing lines...
Lda $dd02
and #$03
sta $dd02
lda $dd00
and #$fc
ora #$02
sta $dd00
And of course I follow with:
lda #$18
ldx #$f8
ldy #$3b
sta $d018
stx $d016
sty $d011
Not known if my image will show...
<a href="http://s48.photobucket.com/user/festeezio/media/koala_zpsxtctbx.." target="_blank"><img src="http://i48.photobucket.com/albums/f245/festeezio/koala_zpsxtctb.." border="0" alt=" photo koala_zpsxtctbxw4.png"/></a>
If it doesn't, here's a direct link..
http://i48.photobucket.com/albums/f245/festeezio/koala_zpsxtctb..
Any suggestions as to what I'm doing wrong?
Image is at $6000. The 2 RAMS mentioned are being read and plotted to the screen/color area. |
| |
soci
Registered: Sep 2003 Posts: 480 |
*= $6000
>6000 pic .binary "a.kla",2
*= $0801
>0801 0b 08 df 07 .word +, 2015
>0805 9e 32 30 36 31 00 .null $9e, ^start
>080b 00 00 + .word 0
.080d a2 00 ldx #$00 start ldx #0
.080f a0 04 ldy #$04 ldy #4
.0811 bd 40 7f lda $7f40,x lp lda pic+8000,x
.0814 9d 00 44 sta $4400,x sta $4400,x
.0817 bd 28 83 lda $8328,x lda pic+9000,x
.081a 9d 00 d8 sta $d800,x sta $d800,x
.081d e8 inx inx
.081e d0 f1 bne $0811 bne lp
.0820 ee 13 08 inc $0813 inc lp+2+range(4)*3
.0823 ee 16 08 inc $0816
.0826 ee 19 08 inc $0819
.0829 ee 1c 08 inc $081c
.082c 88 dey dey
.082d d0 e2 bne $0811 bne lp
.082f 8c 20 d0 sty $d020 sty $d020
.0832 ad 10 87 lda $8710 lda pic+10000
.0835 8d 21 d0 sta $d021 sta $d021
.0838 ad 00 dd lda $dd00 lda $dd00
.083b 29 fc and #$fc and #$fc
.083d 09 02 ora #$02 ora #$02
.083f 8d 00 dd sta $dd00 sta $dd00
.0842 a9 18 lda #$18 lda #$18
.0844 a2 f8 ldx #$f8 ldx #$f8
.0846 a0 3b ldy #$3b ldy #$3b
.0848 8d 18 d0 sta $d018 sta $d018
.084b 8e 16 d0 stx $d016 stx $d016
.084e 8c 11 d0 sty $d011 sty $d011
.0851 4c 51 08 jmp $0851 jmp *
|
| |
The Phantom
Registered: Jan 2004 Posts: 360 |
AHHH!!
Now I see... I'm putting data at $0400, instead of $4400, which became my new screen (as opposed to $0400).
Thanks Soci :D |
| |
The Phantom
Registered: Jan 2004 Posts: 360 |
That was my problem.. I had the bank switch correct, but had my STA at $0400. I just changed my values and son of a bitch if it's not displayed proper....
Thanks Soci :D Now I can get this out today.... |
| |
JackAsser
Registered: Jun 2002 Posts: 2014 |
Quote: why not just store a fixed value to $dd00 though? i dare to say that "properly" ORing the value is very rarely needed - certainly almost never in the usual setup code at the start of your intro/demo/whatever :)
Did "proper" masking for long myself. Such stupidity. My usual setup code is now usually something along:
ldx #$2e
:
lda vic,x
sta $d000,x
dex
bpl :-
vic: .byte register.setup.here...
|
| |
Frantic
Registered: Mar 2003 Posts: 1648 |
Well I also used to do the masking thing for quite a while. :D |
| |
Monte Carlos
Registered: Jun 2004 Posts: 359 |
copy0400: sta src
stx src+1
tsx
lda $0104,x
sta dest
lda $0103,x
sta dest+1
ldx #0
ldy #4
cop:
src = *+1
lda $ffff,x
dest = *+1
sta $ffff,x
inx
bne cop
inc src+2
inc dest+2
dey
bne cop
rts
call with:
lda #<scrn
pha
lda #>scrn
pha
lda #<koala+$1f40
ldx #>koala+$1f40
jsr cop
pla
pla
lda #<$d800
pha
lda #>$d800
pha
lda #<koala+$1f40
ldx #>koala+$1f40
jsr cop
pla
pla
rts
screen and colorram has zero lowbyte, so you may hardcode this in cop routine. but if you want it flexible...
$0100 is the stack beginning. as return address is pushed, you need tsx: lda $0103,x... |
| |
soci
Registered: Sep 2003 Posts: 480 |
I see demand for a separate thread for posting clever routines... |
| |
Oswald
Registered: Apr 2002 Posts: 5094 |
thats overcomplicated, how about:
copy0400:
ldx #0
ldy #4
cop:
src = *+1
lda $ffff,x
dest = *+1
sta $ffff,x
inx
bne cop
inc src+2
inc dest+2
dey
bne cop
rts
call with:
lda #<scrn
sta dest
lda #>scrn
sta dest+1
lda #<koala+$1f40
sta src
lda #>koala+$1f40
sta src+1
jsr copy
lda #<$d800
sta dest
lda #>$d800
sta dest+1
lda #<koala+$1f40
sta src
lda #>koala+$1f40
sta src+1
jsr copy
rts |
| |
Ejner
Registered: Oct 2012 Posts: 43 |
Or no flexibility, just copy what needs to be copied without messing up sprite pointers :-)
ldx #0
copy lda koala+$1f40,x
sta screen,x
lda koala+$2040,x
sta screen+$0100,x
lda koala+$2140,x
sta screen+$0200,x
lda koala+$2228,x
sta screen+$02e8,x
lda koala+$2328,x
sta $d800,x
lda koala+$2428,x
sta $d900,x
lda koala+$2528,x
sta $da00,x
lda koala+$2610,x
sta $dae8,x
inx
bne copy
|
| |
SIDWAVE Account closed
Registered: Apr 2002 Posts: 2238 |
what is this thread ?
we learned to do this in 1985..
thread started by ejner, who dont know me at all, but downvotes my productions. why ejner ?
so now i shitted in your shitty thread...
how to set up a koala pic ?
congrats on showing your codeskills..
how about making some releases and stop downvoting mine ? |
| |
Bitbreaker
Registered: Oct 2002 Posts: 508 |
Ah, Rambones is spreading again love and friendship in the scene. |
| |
Oswald
Registered: Apr 2002 Posts: 5094 |
keep it on topic, here I show Koala Pic
 |
| |
Smasher
Registered: Feb 2003 Posts: 520 |
my routine uses all vic banks and displays 4 koalas...
 |
| |
SIDWAVE Account closed
Registered: Apr 2002 Posts: 2238 |
This is the koala setup i used since 1985.
coded by kaze. he used the book.
TST Ripp #6 |
| |
ChristopherJam
Registered: Aug 2004 Posts: 1409 |
So much cultural appropriation in this thread D''':
Oswald and ZeSmasher better keep their eyes peeled for Dropbears, that's all I can say.
 |
| |
chatGPZ
Registered: Dec 2001 Posts: 11386 |
Koala Interlace, bitch!
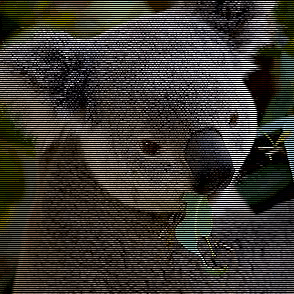 |
| |
Oswald
Registered: Apr 2002 Posts: 5094 |
for bonus points convert it to 16cols :) then the next one make a release named koala pic shower :o) |
| |
SIDWAVE Account closed
Registered: Apr 2002 Posts: 2238 |
;KOALA PIC 2000
lda $dd00
and #%11111100
ora #%00000011
sta $dd00
lda $d016
ora #$10
sta $d016
LDA #$19
STA $D018
lda #$3b
sta $d011 |
| |
Smasher
Registered: Feb 2003 Posts: 520 |
Oswald, I think you cheated... that's drazlace :)
so lemme check aswell: FLI with fli-bug on!
 |
| |
Bitbreaker
Registered: Oct 2002 Posts: 508 |
Quoting SIDWAVE
lda $dd00
and #%11111100
ora #%00000011
sta $dd00
This might break with elite loaders active.
Also, why storing #$19 in $d018?
Where to copy the colram?
Where is $d021 set?
... |